Flutter - Probably needed for every projects
Basic HTTP get, delete
import 'package:http/http.dart' as http;
// get
void _loadItems() async {
final url = Uri.https('abc.asia-southeast1.firebasedatabase.app','table.json');
final response = await http.get(url);
}
// delete
void _removeItem(Model item) {
final url = Uri.https('abc.asia-southeast1.firebasedatabase.app', 'shopping-list/${item.id}.json');
http.delete(url);
}
A simple loading spinner
This may be the simplest loading spinner that we all need to have, right out of the box.
class _SomethingState extends State<Something> {
var _isLoading = true;
void _loadItems() async {
setState(() {
_isLoading = false;
});
}
@override
Widget build(BuildContext context) {
if (_isLoading) {
content = const Center(
child: CircularProgressIndicator(),
);
}
return Scaffold(
body: content
);
}
}
Adding Uuid
// https://pub.dev/packages/uuid/install
flutter pub add uuid
import 'package:uuid/uuid.dart';
const uuid = Uuid();
class Model {
Model({ required this.title}) : id = uuid.v4();
final String id;
final String title;
}
Adding Google fonts and Adding default color
flutter pub add google_fonts
import 'package:google_fonts/google_fonts.dart';
final colorScheme = ColorScheme.fromSeed(
brightness: Brightness.dark,
seedColor: const Color.fromARGB(255, 102, 6, 247),
background: const Color.fromARGB(255, 56, 49, 66),
);
final theme = ThemeData().copyWith(
useMaterial3: true,
scaffoldBackgroundColor: colorScheme.background,
colorScheme: colorScheme,
textTheme: GoogleFonts.ubuntuCondensedTextTheme().copyWith(
titleSmall: GoogleFonts.ubuntuCondensed(
fontWeight: FontWeight.bold,
),
titleMedium: GoogleFonts.ubuntuCondensed(
fontWeight: FontWeight.bold,
),
titleLarge: GoogleFonts.ubuntuCondensed(
fontWeight: FontWeight.bold,
),
),
);
Example Global State using Riverpod
flutter pub add flutter_riverpod
---
import 'package:flutter_riverpod/flutter_riverpod.dart';
import 'package:favorite_places/providers/user_places.dart';
// example create lib/providers/user_places.dart
import 'package:flutter_riverpod/flutter_riverpod.dart';
import 'package:favorite_places/models/place.dart';
class UserPlacesNotifier extends StateNotifier<List<Place>> {
UserPlacesNotifier() : super(const []);
void addPlace(String title) {
final newPlace = Place(title: title);
state = [newPlace, ...state];
}
}
final userPlacesProvider = StateNotifierProvider<UserPlacesNotifier, List<Place>>(
(ref) => UserPlacesNotifier());
Modifying main.dart and those that are from the stateful widget and stateless widget
// wrap MyApp using ProviderScope in main.dart
import 'package:flutter_riverpod/flutter_riverpod.dart';
void main() {
runApp(
const ProviderScope(child: const MyApp()),
);
}
// screen that wants to use the global state from stateful widget
// update the extends
from
class AddPlaceScreen extends StatefulWidget {
to
class AddPlaceScreen extends ConsumerStatefulWidget {
and
from
State<AddPlaceScreen> createState() => _AddPlaceScreenState();
to
ConsumerState<AddPlaceScreen> createState() => _AddPlaceScreenState();
and
from
class _AddPlaceScreenState extends State<AddPlaceScreen> {
to
class _AddPlaceScreenState extends ConsumerState<AddPlaceScreen> {
---
// to use function in global state
ref.read(userPlacesProvider.notifier).addPlace(enteredTitle);
Using Image Picker
image_picker | Flutter package
Flutter plugin for selecting images from the Android and iOS image library, and taking new pictures with the camera.
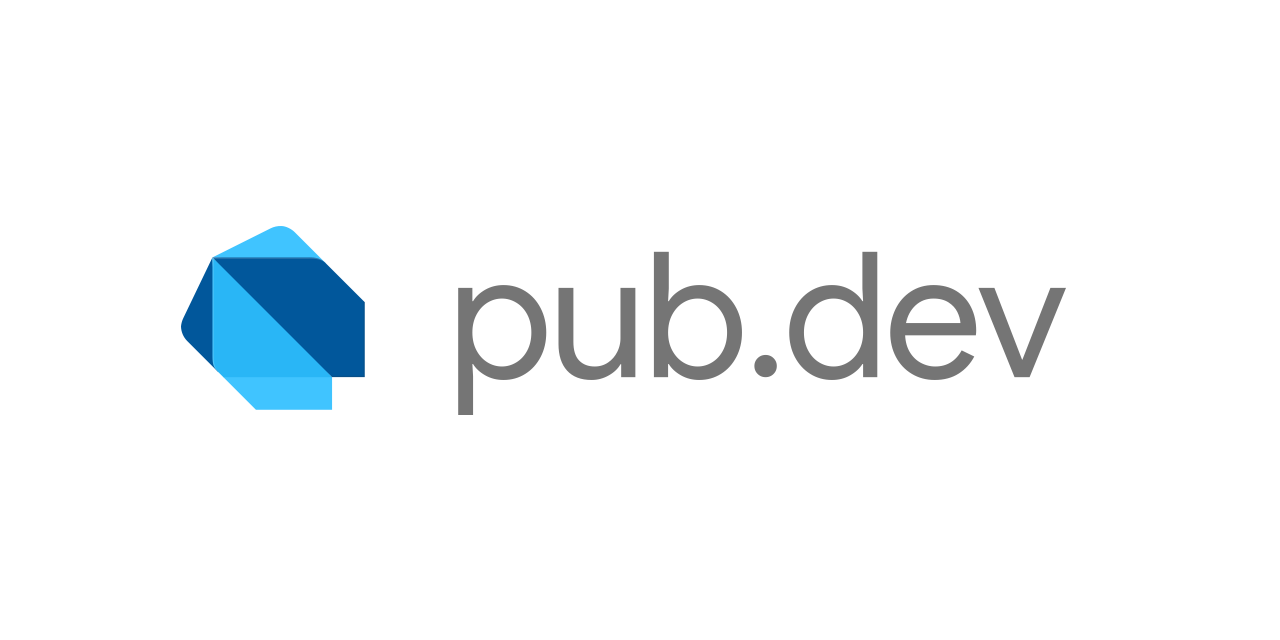
flutter pub add image_picker
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:image_picker/image_picker.dart';
class ImageInput extends StatefulWidget {
const ImageInput({super.key});
@override
State<ImageInput> createState() => _ImageInputState();
}
class _ImageInputState extends State<ImageInput> {
File? _selectedImage;
void _takePicture() async {
final imagePicker = ImagePicker();
final pickedImage =
await imagePicker.pickImage(source: ImageSource.camera, maxWidth: 600);
if (pickedImage == null) {
return;
}
setState(() {
_selectedImage = File(pickedImage.path);
});
}
@override
Widget build(BuildContext context) {
Widget content = TextButton.icon(
icon: const Icon(Icons.camera),
label: const Text('Take Picture'),
onPressed: _takePicture,
);
if (_selectedImage != null) {
content = GestureDetector(
onTap: _takePicture,
child: Image.file(_selectedImage!,
fit: BoxFit.cover, width: double.infinity, height: double.infinity),
);
}
return Container(
decoration: BoxDecoration(
border: Border.all(
width: 1,
color: Theme.of(context).colorScheme.primary.withOpacity(0.2))),
height: 250,
width: double.infinity,
child: content,
);
}
}
Changing minSdk for Android
// local.properties
flutter.minSdkVersion=23
// From
From : minSdkVersion flutter.minSdkVersion
// Tp
minSdkVersion localProperties.getProperty('flutter.minSdkVersion').toInteger()
Location
location | Flutter package
Cross-platform plugin for easy access to device’s location in real-time.
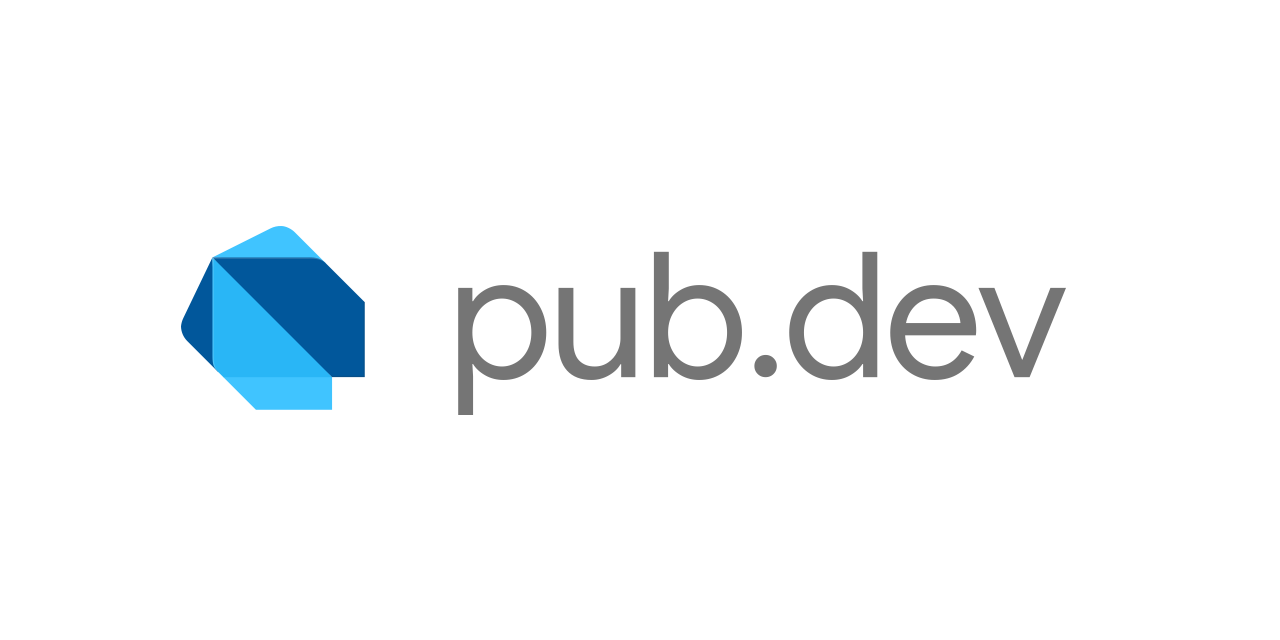
flutter pub add location
import 'package:flutter/material.dart';
import 'package:location/location.dart';
class LocationInput extends StatefulWidget {
const LocationInput({super.key});
@override
State<LocationInput> createState() => _LocationInputState();
}
class _LocationInputState extends State<LocationInput> {
Location? _pickedLocation;
var _isGettingLocation = false;
void _getCurrentLocation() async {
Location location = Location();
bool serviceEnabled;
PermissionStatus permissionGranted;
LocationData locationData;
serviceEnabled = await location.serviceEnabled();
if (!serviceEnabled) {
serviceEnabled = await location.requestService();
if (!serviceEnabled) {
return;
}
}
permissionGranted = await location.hasPermission();
if (permissionGranted == PermissionStatus.denied) {
permissionGranted = await location.requestPermission();
if (permissionGranted != PermissionStatus.granted) {
return;
}
}
setState(() {
_isGettingLocation = true;
});
locationData = await location.getLocation();
setState(() {
_isGettingLocation = false;
});
print(locationData.latitude);
print(locationData.longitude);
}
@override
Widget build(BuildContext context) {
Widget previewContent = Text(
'No location chosen',
textAlign: TextAlign.center,
style: Theme.of(context).textTheme.bodyLarge!.copyWith(
color: Theme.of(context).colorScheme.onBackground,
),
);
if (_isGettingLocation) {
previewContent = const CircularProgressIndicator();
}
return Column(
children: [
Container(
height: 170,
width: double.infinity,
decoration: BoxDecoration(
border: Border.all(
width: 1,
color:
Theme.of(context).colorScheme.primary.withOpacity(0.2))),
child: previewContent,
),
Row(
children: [
TextButton.icon(
icon: const Icon(Icons.location_on),
label: const Text('Get Current Location'),
onPressed: _getCurrentLocation,
),
TextButton.icon(
icon: const Icon(Icons.map),
label: const Text('Select on Map'),
onPressed: () {},
),
],
),
],
);
}
}
Icons Launcher
flutter_launcher_icons | Dart package
A package which simplifies the task of updating your Flutter app’s launcher icon.
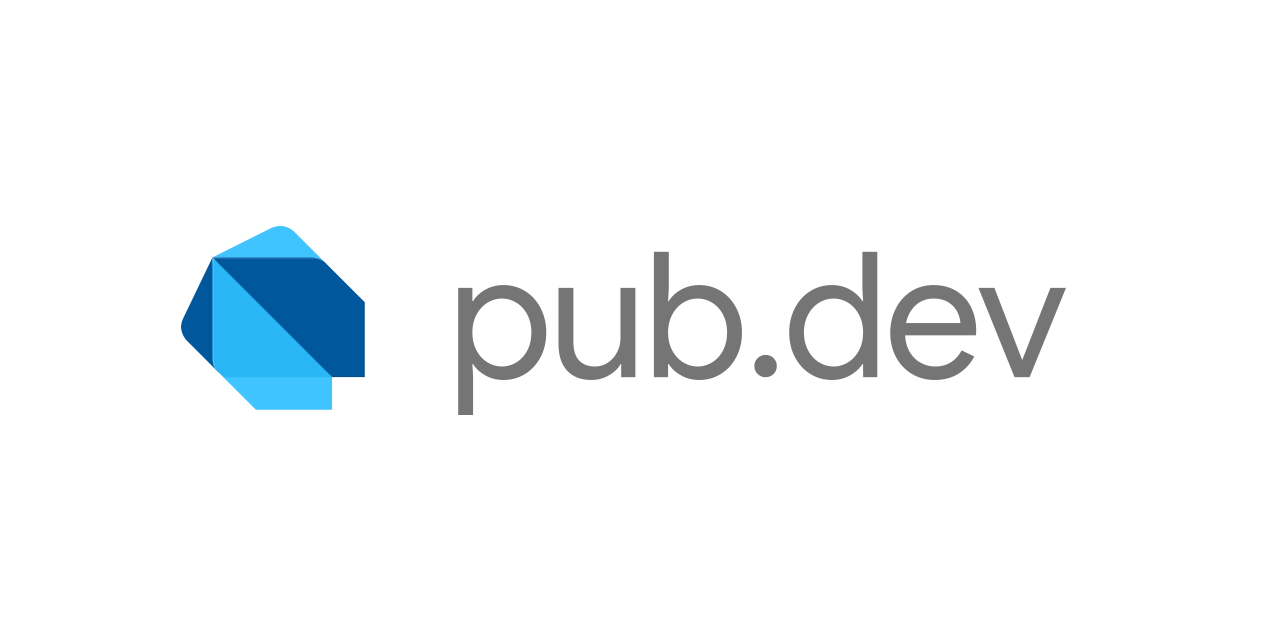
flutter pub add flutter_launcher_icons
# pubspec.yaml
flutter_launcher_icons:
android: "launcher_icon"
ios: false
image_path: "assets/images/icon.png"
min_sdk_android: 20 # android min sdk min:16, default 21
# terminal
flutter clean
flutter pub get
flutter pub run flutter_launcher_icons
Flutter Webview Pro
There was a difference on Webview webview_flutter, where I cannot show file upload like <input type="file"> this, or maybe I have added the permission but when I use this and add...
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.CAMERA"/>
flutter_webview_pro | Flutter package
A Flutter plugin that provides a WebView widget who Support photo upload/take camera and Geolocation.
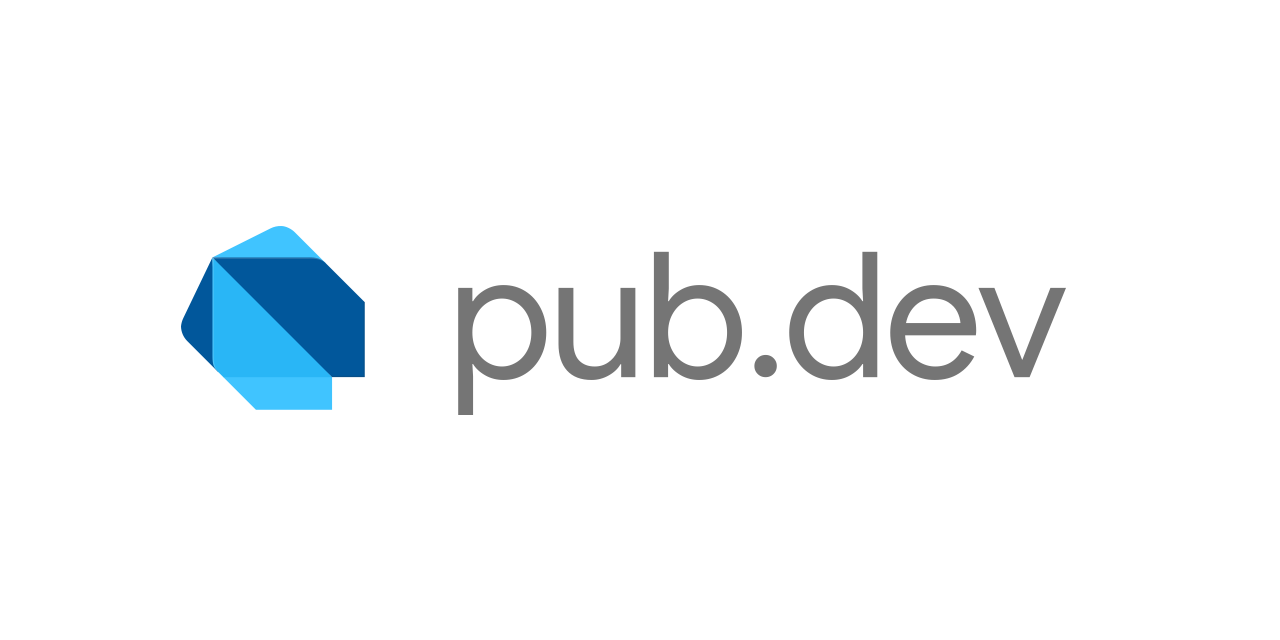
import 'package:flutter_webview_pro/webview_flutter.dart';
@override
void initState() {
super.initState();
if (Platform.isAndroid) {
WebView.platform = SurfaceAndroidWebView();
}
}
@override
Widget build(BuildContext context) {
return const Scaffold(
body: WebView(
initialUrl: 'https://website.com/webview/worker/login',
javascriptMode: JavascriptMode.unrestricted,
),
);
}