Stripe API laravel
Finally got a chance to try out stripe, in Indonesia, we don't use Stripe that much because it uses a different currency and most of my client uses IDR, not USD.
Finally got a chance to try out stripe, in Indonesia, we don't use Stripe that much because it uses a different currency and most of my client uses IDR, not USD.
Form #1 Manual
composer require stripe/stripe-php
<form
role="form"
action="{{ route('payment.stripe') }}"
method="post"
class="require-validation"
data-cc-on-file="false"
data-stripe-publishable-key="{{ env('STRIPE_KEY') }}"
id="payment-form">
@csrf
<input
autocomplete='off' class='card-number mt-1 block w-full py-2 px-4 border-2 border-gray-200 rounded-md mb-3'
type='text' placeholder="Enter your card number" maxlength="16">
<div class="flex gap-3">
<input autocomplete='off'
class='card-cvc mt-1 block w-full py-2 px-4 border-2 border-gray-200 rounded-md mb-3' placeholder='ex. 311'
type='text' placeholder="CVC" maxlength="3">
<input
class='card-expiry-month mt-1 block w-full py-2 px-4 border-2 border-gray-200 rounded-md mb-3' placeholder='MM'
type='text' placeholder="Expiration Month" maxlength="2">
<input
class='card-expiry-year mt-1 block w-full py-2 px-4 border-2 border-gray-200 rounded-md mb-3' placeholder='YYYY'
type='text' placeholder="Expiration Year" maxlength="4">
</div>
<div class='form-row row'>
<div class='col-md-12 error form-group hidden text-red-500'>
<div class='alert-danger alert'>Please correct the errors and try
again.</div>
</div>
</div>
<div class="row">
<div class="col-xs-12">
<button class="py-2 px-5 text-yellow-500 font-bold bg-white rounded-2xl uppercase text-sm border-2 border-yellow-500 hover:bg-yellow-500 hover:text-white w-full block text-center mt-5" type="submit">Pay Now</button>
</div>
</div>
<script type="text/javascript" src="https://js.stripe.com/v2/"></script>
<script type="text/javascript">
$(function() {
var $form = $(".require-validation");
$('form.require-validation').bind('submit', function(e) {
var $form = $(".require-validation"),
inputSelector = ['input[type=email]', 'input[type=password]',
'input[type=text]', 'input[type=file]',
'textarea'].join(', '),
$inputs = $form.find('.required').find(inputSelector),
$errorMessage = $form.find('div.error'),
valid = true;
$errorMessage.addClass('hidden');
$('.has-error').removeClass('has-error');
$inputs.each(function(i, el) {
var $input = $(el);
if ($input.val() === '') {
$input.parent().addClass('has-error');
$errorMessage.removeClass('hidden');
e.preventDefault();
}
});
if (!$form.data('cc-on-file')) {
e.preventDefault();
Stripe.setPublishableKey($form.data('stripe-publishable-key'));
Stripe.createToken({
number: $('.card-number').val(),
cvc: $('.card-cvc').val(),
exp_month: $('.card-expiry-month').val(),
exp_year: $('.card-expiry-year').val()
}, stripeResponseHandler);
}
});
function stripeResponseHandler(status, response) {
if (response.error) {
$('.error')
.removeClass('hidden')
.find('.alert')
.text(response.error.message);
} else {
/* token contains id, last4, and card type */
var token = response['id'];
$form.find('input[type=text]').empty();
$form.append("<input type='hidden' name='stripeToken' value='" + token + "'/>");
$form.get(0).submit();
}
}
});
</script>
\Stripe\Stripe::setApiKey(env('STRIPE_SECRET'));
\Stripe\Charge::create ([
"amount" => (int)( ($nominal + ($nominal * 0.029) + 0.3) * 100),
"currency" => "usd",
"source" => $request->stripeToken,
"description" => "Payment From User - ". auth()->user()->name
]);
Stripe CLI
Getting payment intend
This is needed to check the payment intend when a user successfully paid to your server, this also helped with local development which helps me a lot. I use laravel so I made a route in api, if I were to use in route web it shows error 419, I looked around you can add an exception in verifyCsrf but for me didn't work so I just put in api route.
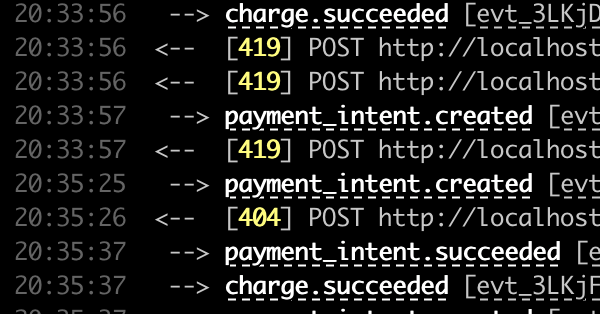
Route::post('/payment/stripe/webhook', [PaymentController::class, 'stripe_webhook'])->name('payment.stripe.webhook');
to run this
stripe login
stripe listen --forward-to localhost:8000/api/payment/stripe/webhook
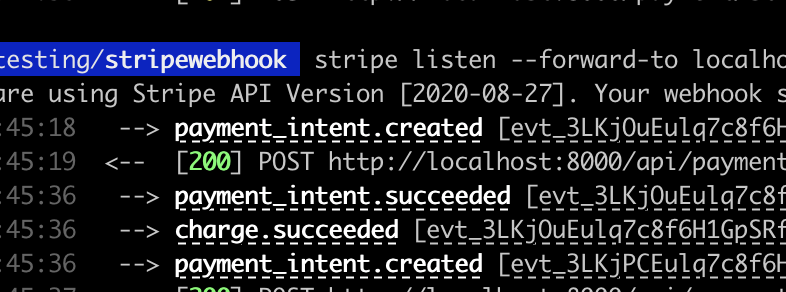